What the heck is defer?
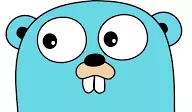
Do you struggle to get the hang of defer
too?
Worry not 😄, I will try to explain it as simply as I can in this post.
What is defer?
A defer statement defers the execution of a function until the surrounding function returns.
The deferred call’s arguments are evaluated immediately, but the function call is not executed until the surrounding function returns.
This program explains the execution of defer
in a bit easier way:
|
|
Output:
|
|
Tip
Deferred function calls are pushed onto a stack.
When a function returns, its deferred calls are executed in last-in-first-out order.
Here is a small program which visualises this:
|
|
Output:
|
|
However remember deferred function’s arguments are evaluated immediately, hence it can give a different result
|
|
A particularly good use of defer
is to close a file.
|
|
Abstract
Deferring a call to a function such as Close has two advantages. First, it guarantees that you will never forget to close the file, a mistake that’s easy to make if you later edit the function to add a new return path.
Second, it means that the close sits near the open, which is much clearer than placing it at the end of the function.
Source
Tip
Read an in-depth article on
defer
to understand it better: Defer, Panic, and Recover